6/28 공부 내용 정리
1. 쿠다(CUDA)는 NVIDIA가 개발한 병렬 컴퓨팅 플랫폼이다. 이는 그래픽 처리 장치(GPU)를 사용하여 고성능 병렬 컴퓨팅을 수행하는 데 사용된다. 쿠다를 사용하면 GPU의 수천 개의 코어를 활용하여 동시에 여러 작업을 처리할 수 있다. 이를 통해 대량의 데이터를 동시에 처리하거나 복잡한 계산 작업을 빠르게 수행할 수 있다.
2. 파이토치(PyTorch) 프레임워크 역사
1) 2007년 Theano 시작
2) 2013년 Caffe (Caffe2)
3) 2015년 tensorflow
- DL Framework 전환점이 되는 시기
4) 2016년 CNTK
5) 2016년 Keras 1.0 출시
파이썬 코드
3. Colab에서 GPU를 사용해 보자.
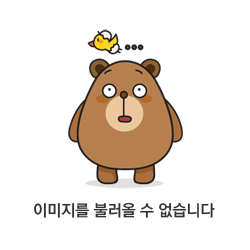
4. 실행하면 false가 나온다. CPU를 사용하고 있기 때문이다.
data = [
[1, 2],
[3, 4]
]
x = torch.tensor(data)
print(x.is_cuda)
5. GPU로 옮겨보자. 결과는 True로 나온다. 하지만 다시 CPU로 옮기면 False가 나온다.
data = [
[1, 2],
[3, 4]
]
x = torch.tensor(data)
print(x.is_cuda)
#GPU로 옮기기 1
x = x.cuda()
print(x.is_cuda)
#GPU로 옮기기 2
device = torch.device("cuda" if torch.cuda.is_available() else "cpu")
x = x.to(device)
print(x.is_cuda)
#CPU로 옮기기 1
x = x.cpu()
print(x.is_cuda)
6. 현재 상태를 확인해 보자.
tensor = torch.rand(3,4)
print(tensor)
print(f"shape : {tensor.shape}")
print(f"Data type: {tensor.dtype}")
print(f"Device: {tensor.device}")
결과
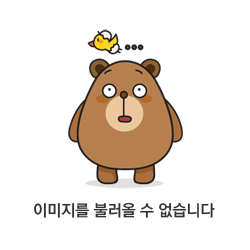
7. 장치가 다르면 연산 오류가 발생한다.
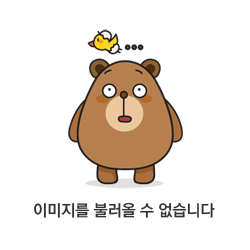
Expected all tensors to be on the same device, but found at least two devices, cuda:0 and cpu! (when checking argument for argument mat2 in method wrapper_CUDA_mm)
8. .cpu()로 장치를 바꿔줘야 오류가 안남. 행렬 곱셈이 진행됨.
# GPU 장치의 텐서
a = torch.tensor([
[1, 1],
[2, 2]
]).cuda()
# CPU 장치의 텐서
b = torch.tensor([
[6, 7],
[8, 9]
])
# print(torch.matmul(a, b)) # 오류 발생
print(torch.matmul(a.cpu(), b))
9. 파이참에 파이토치를 설치하고 실행.
import torch
a = torch.tensor([1, 2, 3])
b = torch.tensor([4, 5, 6])
c = a + b
print(c)
print(c.is_cuda)
print(torch.tensor([[1, 2], [3, 4]]))
10. 연습
#Numpy 배열에서 텐서를 초기화
c = (a + b).numpy()
print(c)
print(type(c))
result = c * 10
tensor = torch.from_numpy(result)
print(tensor)
print(type(tensor))
print("========================================")
# 다른 텐서로부터 텐서 초기화
x= torch.tensor([
[5, 7],
[1, 2]
])
# x와 같은 모양과 자료형을 가짐. 값이 1인 텐서 생성
x_ones = torch.ones_like(x)
print(x_ones)
# x와 같은 모양이지만, 자료형은 float32로 덮어씀. 값은 random.
x_rand = torch.rand_like(x, dtype=torch.float32)
print(x_rand)
print("========================================")
# 텐서의 특정 차원 접근하기
tensor = torch.tensor([
[1, 2, 3, 4],
[5, 6, 7, 8],
[9, 10, 11, 12]
])
print(tensor.shape)
print(tensor[0]) # first row
print(tensor[:, 0]) # firt column
print(tensor[:, -1]) # last column
# 두 텐서를 이어 붙여 연결
print("========================================")
# dim : 텐서를 이어 붙이기 위한 축
# 0번 축(행)
result1 = torch.cat([tensor, tensor, tensor], dim=0)
print(result1)
result2 = torch.cat([tensor, tensor, tensor], dim=1)
print(result2)
11. Fashion-MNIST 자료 다운로드
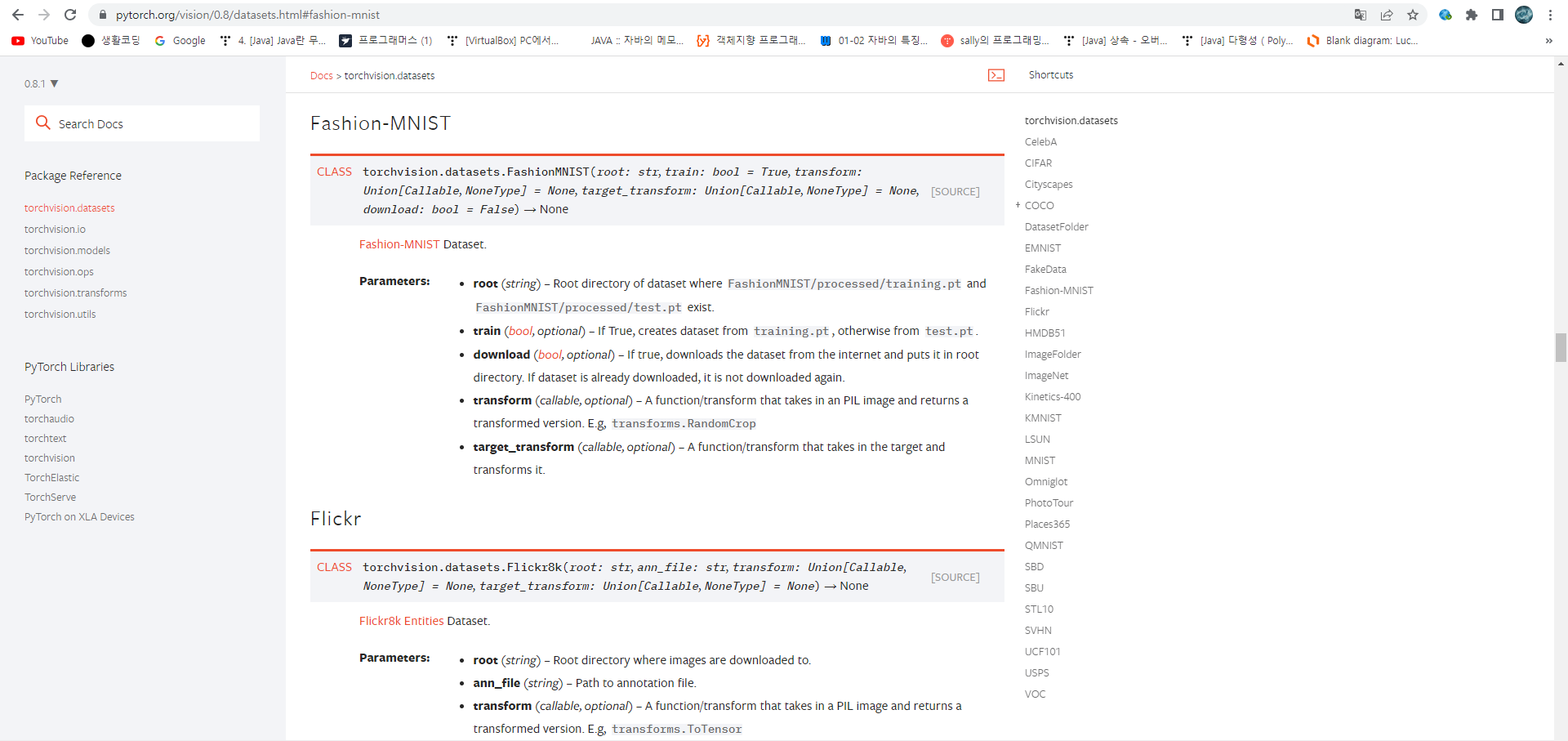
12. Fashion-MNIST data 불러오고, 데이터 시각화하기
import torch
from torch import nn
from torch.utils.data import DataLoader, Dataset
from torchvision import datasets
import torchvision.transforms as tr
from torchvision.transforms import ToTensor
import matplotlib.pyplot as plt
import numpy as np
'''
* torchvision
- 파이토치에서 제공하는 데이터 셋들이 모여있는 패키지
- Fashion-MNIST data
* DataLoader
- Dataset을 model에 공급할 수 있도록 iterable 객체로 감싸주는 클래스
* 신경망 구축 및 실행순서
1) Fashion-MNIST data 불러오기
2) 데이터 시각화 *** 여기까지 완료했음
3) DataLoader 만들기
4) Model Class 만들기
5) Training / Validation Function
'''
training_data = datasets.FashionMNIST(
root="data", # 저장할 디렉토리 지정
train=True, # 훈련데이터 로드
download=True, # 데이터셋을 인터넷에서 다운로드 지정
transform=ToTensor() # 이미지를 PyTorch의 텐서로 변환하는 함수
)
print(training_data)
print(type(training_data))
print("-" * 50)
print(training_data[0])
# 데이터 시각화
labels_map = {
0: "T-shirt/top",
1: "Trouser",
2: "Pullover",
3: "Dress",
4: "Coat",
5: "Sandal",
6: "Shirt",
7: "Sneaker",
8: "Bag",
9: "Ankle boot"
}
figure = plt.figure(figsize=(8, 8))
cols, rows = 3, 3
for i in range(1, cols * rows + 1):
sample_idx = torch.randint(len(training_data), size=(1,)).item()
img, label = training_data[sample_idx]
figure.add_subplot(rows, cols, i)
plt.title(labels_map[label])
plt.axis("off")
plt.imshow(img.squeeze(), cmap="gray") # 텐서의 크기에서 차원의 크기가 1인 것을 제거.
plt.show()
'코딩 학원(국비지원)' 카테고리의 다른 글
코딩 국비지원 학원 6개월, 일경험 3개월 이후 나의 커리어 (6) | 2024.11.04 |
---|---|
국비교육(지능형 웹서비스 풀스택(프런트엔드, 백엔드) 개발 훈련과정) 수료식 (1) | 2023.07.01 |
108일차 코딩학원 (Tensorflow, @tf.function) (0) | 2023.06.27 |
107일차 코딩학원 (1) | 2023.06.26 |
활성(Activation)함수 그래프 그리기. (106일차 코딩학원) (2) | 2023.06.23 |